How to Avoid a JavaScript Mess
At Kscope18, I presented How to Avoid a JavaScript Mess at the #LetsWreckThisTogether talks. Here it is, turned into a blog post.
We all want to write clean code.
I was attending Mike Hichwa's session at Kscope18's Sunday symposium, and he revealed numbers about language usage within the frameworks we use.
APEX is made up of over a million lines of JS.
ORDS contains over 300 000 lines of JS.
And I keep hearing we shouldn't do JavaScript in APEX. That sounds counter intuitive to me. So instead of hiding from JavaScript, maybe we should just embrace it.
But when you start doing more JavaScript, it is easy to fall into the culprits of the language. One important challenge you'll face when developing a large scale application, is keeping your client-side codebase from becoming a huge mess.
The 4 tips below are my kick-start for a cleaner JavaScript usage in APEX.
Tip #1: Keep It Out of APEX
In all my years of APEX development, I found that APEX is best used when the least amount of code is stored in the application itself.
It's common practice to wrap PL/SQL code in packages and SQL into views. Once we have all that business logic in the database, all APEX should be doing is referencing those objects. It's easier to manage and it's easier to version control.
For some reason, when writing JavaScript, developers tend to forget these good intentions and good practices.
One lazy excuse I hear too often is:
I just have to write a single line of JavaScript, so it’s not worth the hassle of creating a file for it.
Let's be real, that's never the case. My point is: there should not be a single line of JavaScript business logic within APEX. Only invoke functions from external files.
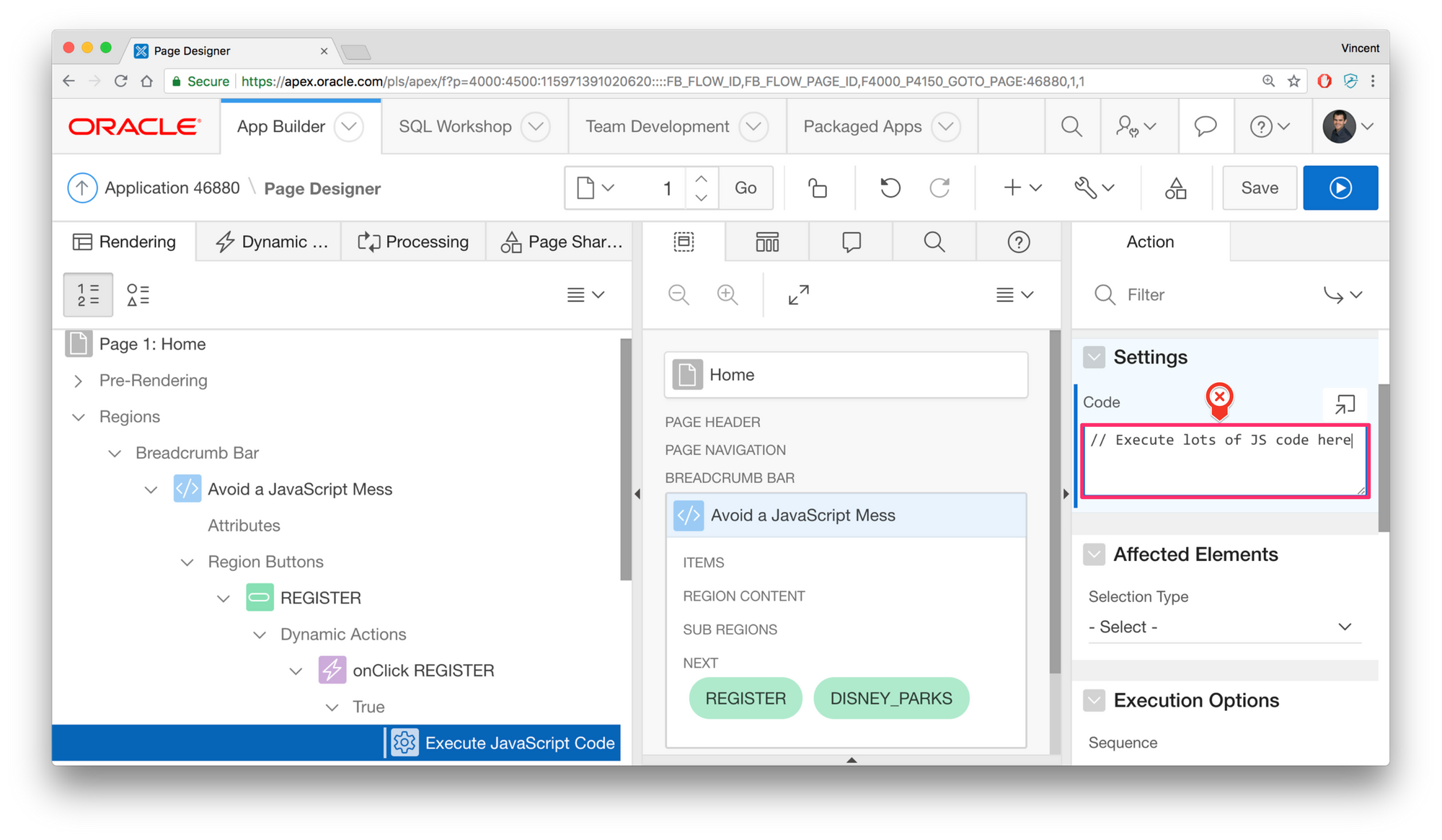
Let’s look at the above. Observations:
- We have a simple button
- It has a dynamic action attached to its onClick event
- Dynamic Action triggers an action of type: Execute JavaScript code
So we might be tempted to put the JavaScript logic on the righthand side, but that’s wrong!
Instead, what we want is moving business logic JavaScript code into a file, and wrap it inside of a function, like the following top level function named registerKscope()
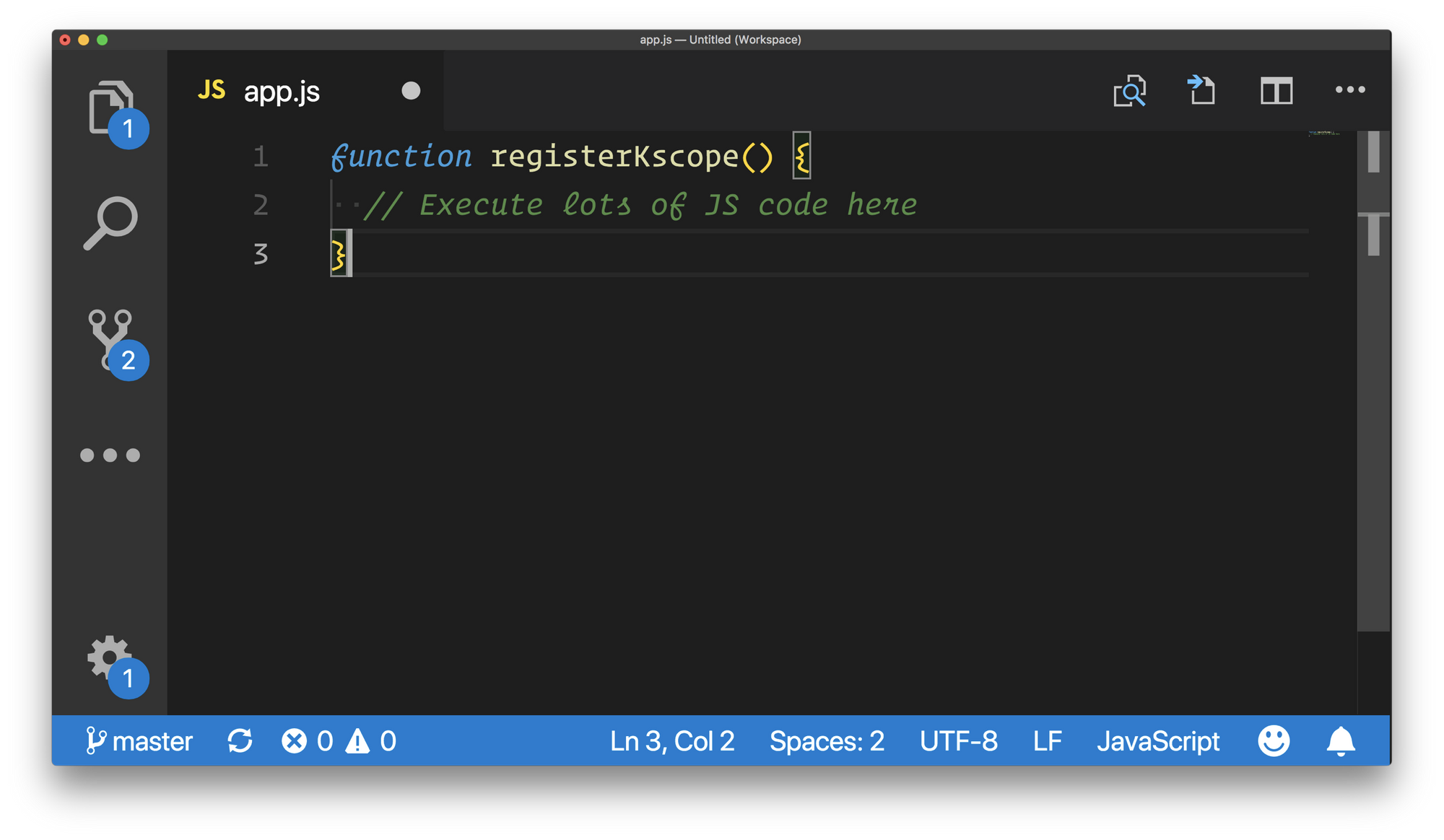
Then, in page designer we can invoke function registerKscope()
.
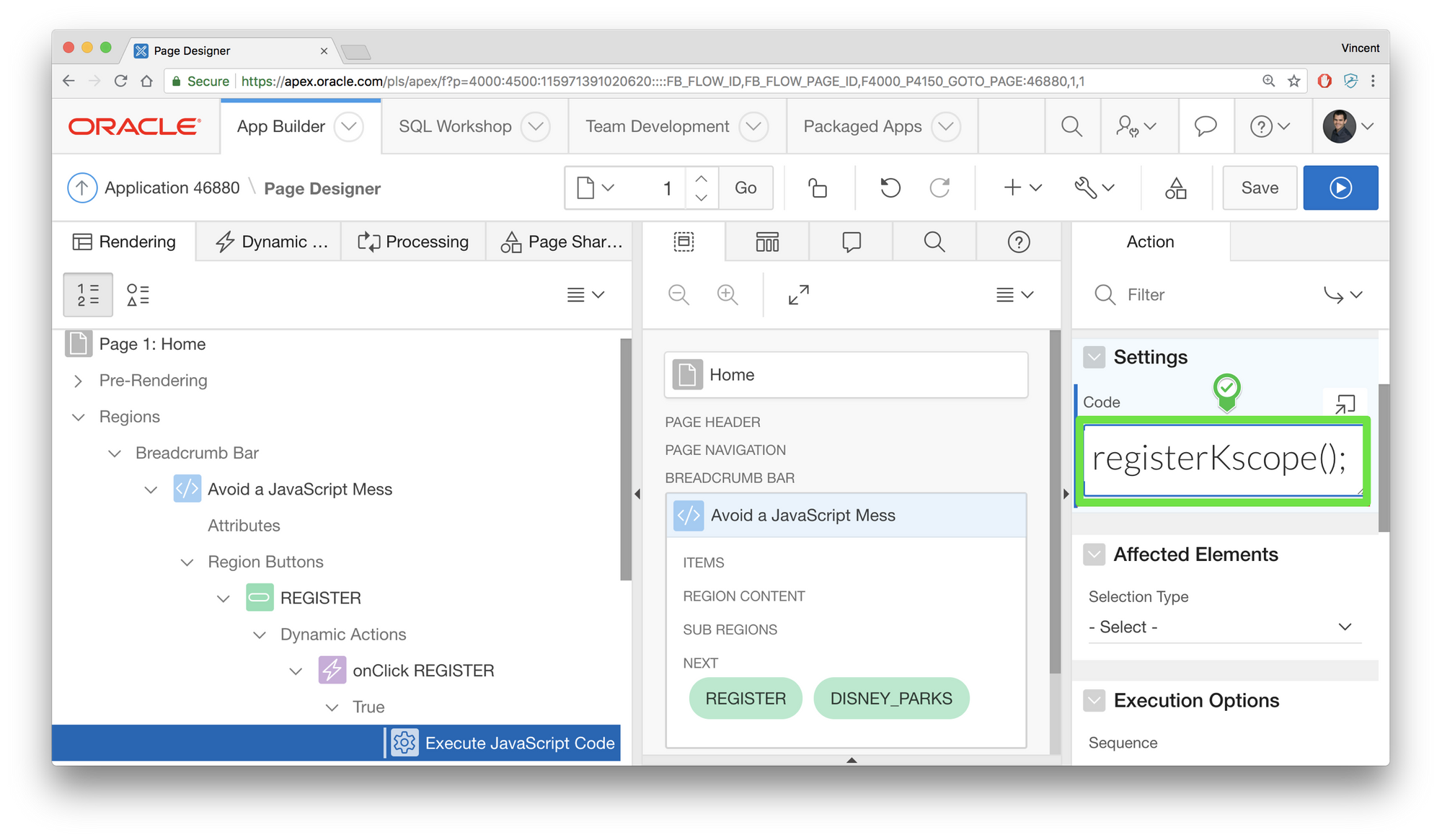
TLDR:
- APEX is best used when the least amount of code is stored in the application
- We constantly wrap PL/SQL into packages and SQL into views. JavaScript can do it too.
- Lazy excuse: "I only have to write one line of JavaScript"
- APEX should only invoke JavaScript functions coming from external files
Tip #2: Modularize your JavaScript
What's the purpose of a PL/SQL package? Giving context to a function or a procedure. So when invoking a PL/SQL API, we would use <package_name>.<procedure_name>
.
Similarly, JavaScript can be modularized with namespaces, and it can even go deeper than two levels. The whole APEX JavaScript API is built like this. Example:
/**
* @namespace odtug
**/
var odtug = odtug || {};
/**
* @module kscope18
**/
odtug.kscope18 = {
/**
* @function register
* @example odtug.kscope18.register();
**/
register: function () {
// Insert JavaScript here
}
};
Having such a structure in your functions makes your files MUCH cleaner.
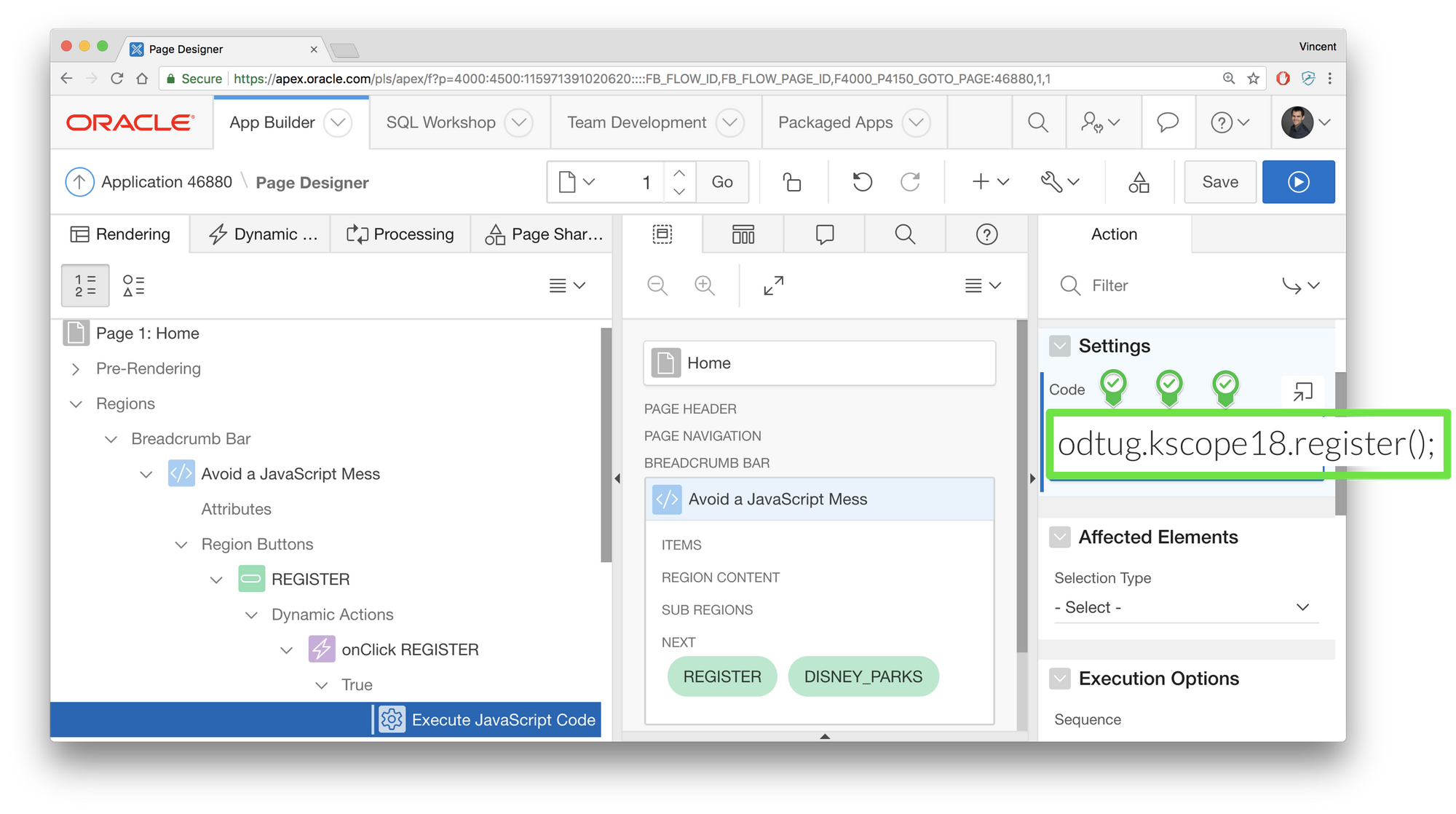
If you are building a large scale application, a great pattern would be to have one JavaScript file per APEX page, each file representing a JavaScript module for your application. Example: myApp.p1.doSomething();
and myApp.p2.doSomethingElse();
TLDR:
- A namespace gives context to JavaScript functions, similarly to PL/SQL packages
- Namespaces allow building JavaScript APIs with more than two levels (Example:
apex.server.process
)- Makes files much cleaner
- Pattern idea: one JavaScript module per APEX page (Example:
myApp.p1.doSomething();
andmyApp.p2.doSomethingElse();
)
Tip #3: Use a Modern Code Editor
There are so many good code editors today. I think it's fair to say that the most popular ones are (in no particular order)
Why would you want to use them? Here's an important rundown:
- IntelliSense: is a term referring to a variety of code features like code completion and parameter information.
- Multi-cursor editing: Why make one change at a time when you can make ten? This feature alone should be enough to persuade you to switch.
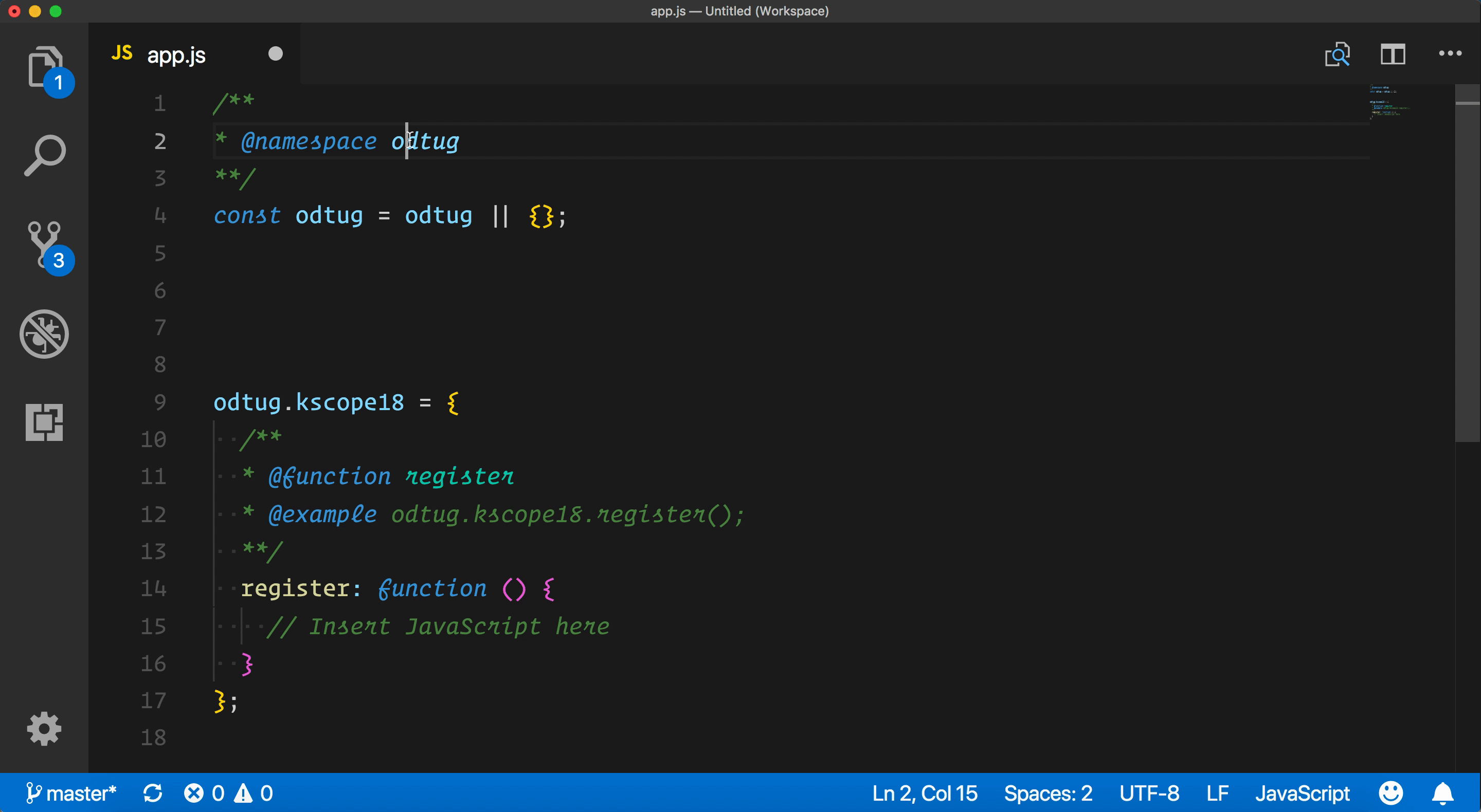
- Plugins: These editors have rich plugin ecosystems. The editor's out-of-the-box features are just the start. Plugins let you add new languages, code linters, text transformations and way more. Anything you can think of applying to your code, there’s a plugin for that.
- Command palette: Being efficient at keyboard shortcuts is important, but if you can just remember the shortcut for the command palette, then you have every possible action at your fingertips, through a searchable list popup.
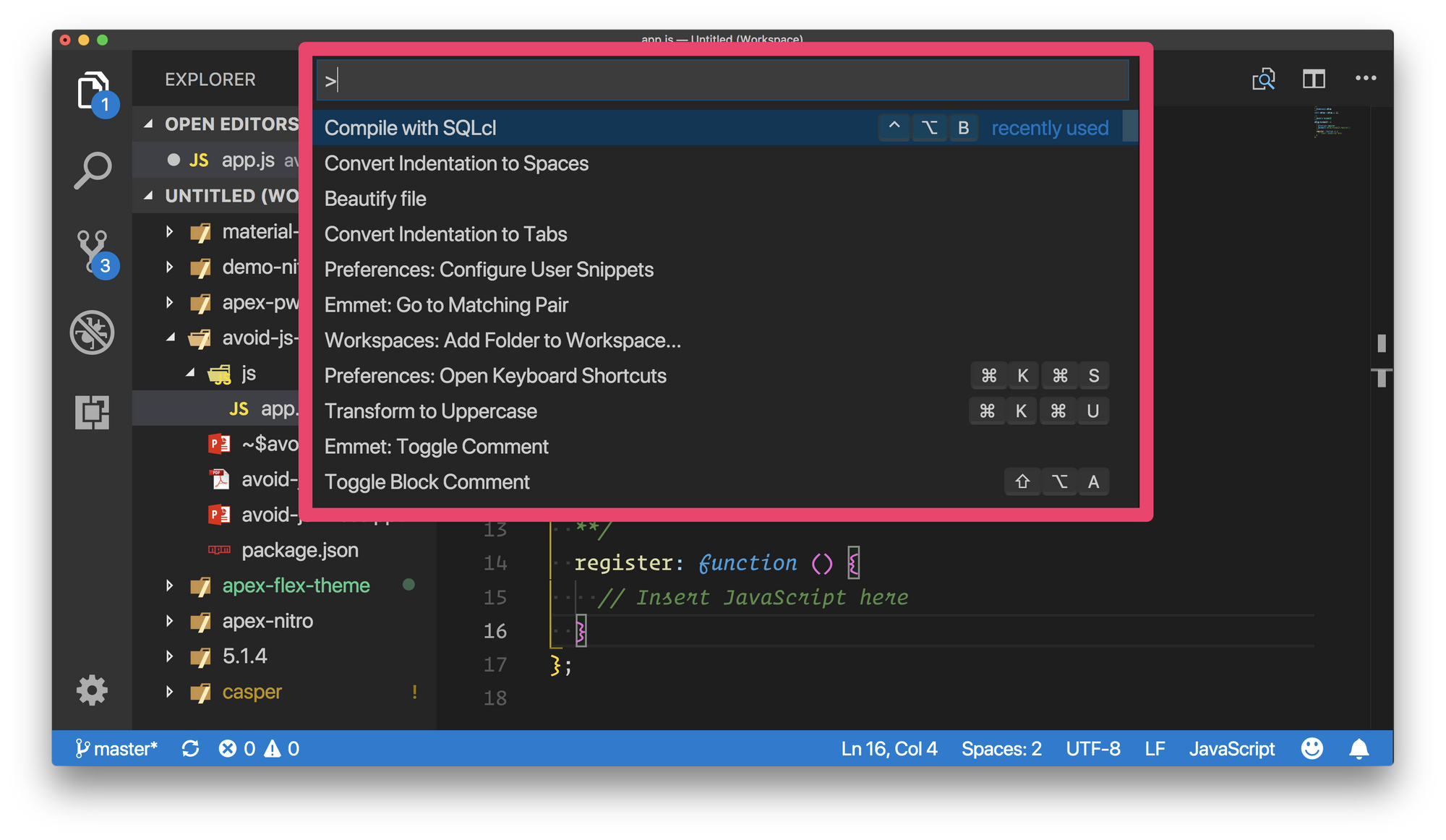
- Snippets: Some of them are built in, like JavaScript's
forEach
or otherwise you can add your own APEX Snippets (apex.server.process
)
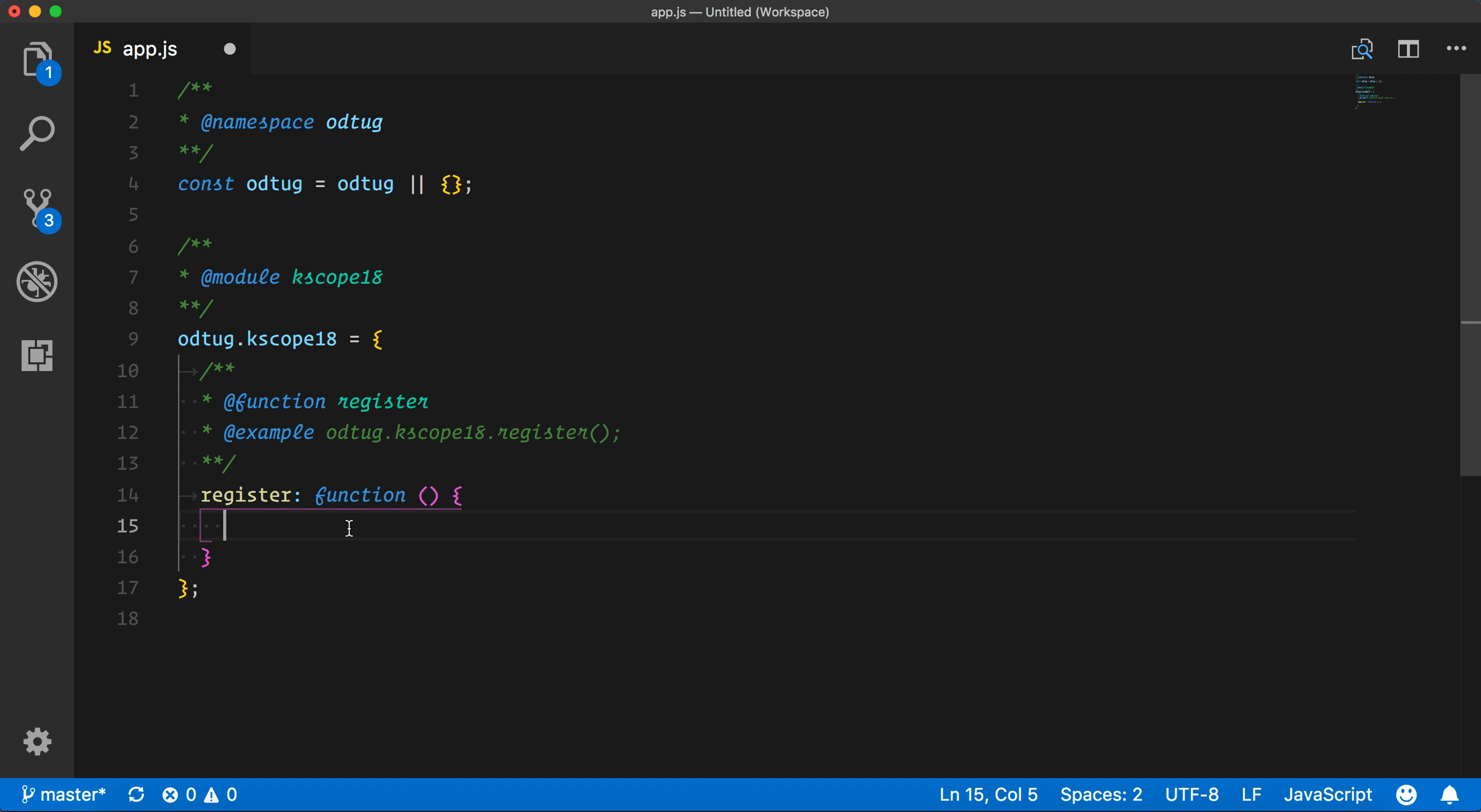
Non-JavaScript bonus feature: it compiles against the Oracle database too! Thanks to a plugin by Trent Schafer
Being comfortable with a new code editor does take time, but don't be discouraged by the first few days. The productivity benefits are worth it.
TLDR:
- IntelliSense: JavaScript code completion
- They've got a plugin for everything
- Command palette: one shortcut to access all available actions
- Baked with premade JavaScript code snippets (
if
statement,loop
statement, etc.)- Custom code snippets (your own snippets, APEX snippets perhaps?)
Tip #4: Use a Style Linter
JavaScript is the perfect language to give up your personal coding style habits. A style linter is a tool that reads your file and detects coding style anomalies.
I am using XO, and it mercilessly tells me where my coding style is inconsistent. We should not spend any time developing and maintaining our own coding style. Let's hand that over to linters.
To use XO in my project directory, I would simply do:
xo
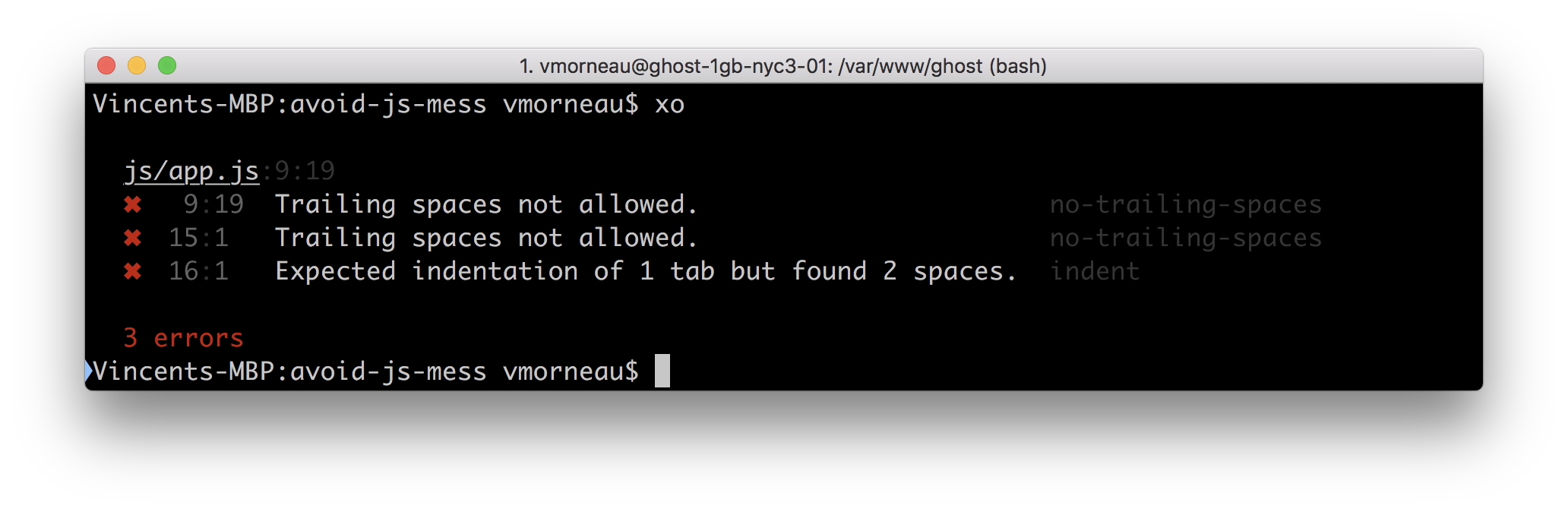
Or even better, if I want XO to fix my code automatically, I can do:
xo --fix
Needless to say, there is more to writing clean code than these 4 tips, so this blog post is subject to be updated in the future.
Have any insights for writing cleaner JavaScript in APEX? Let me know in the comments.