Using APEX Javascript Events
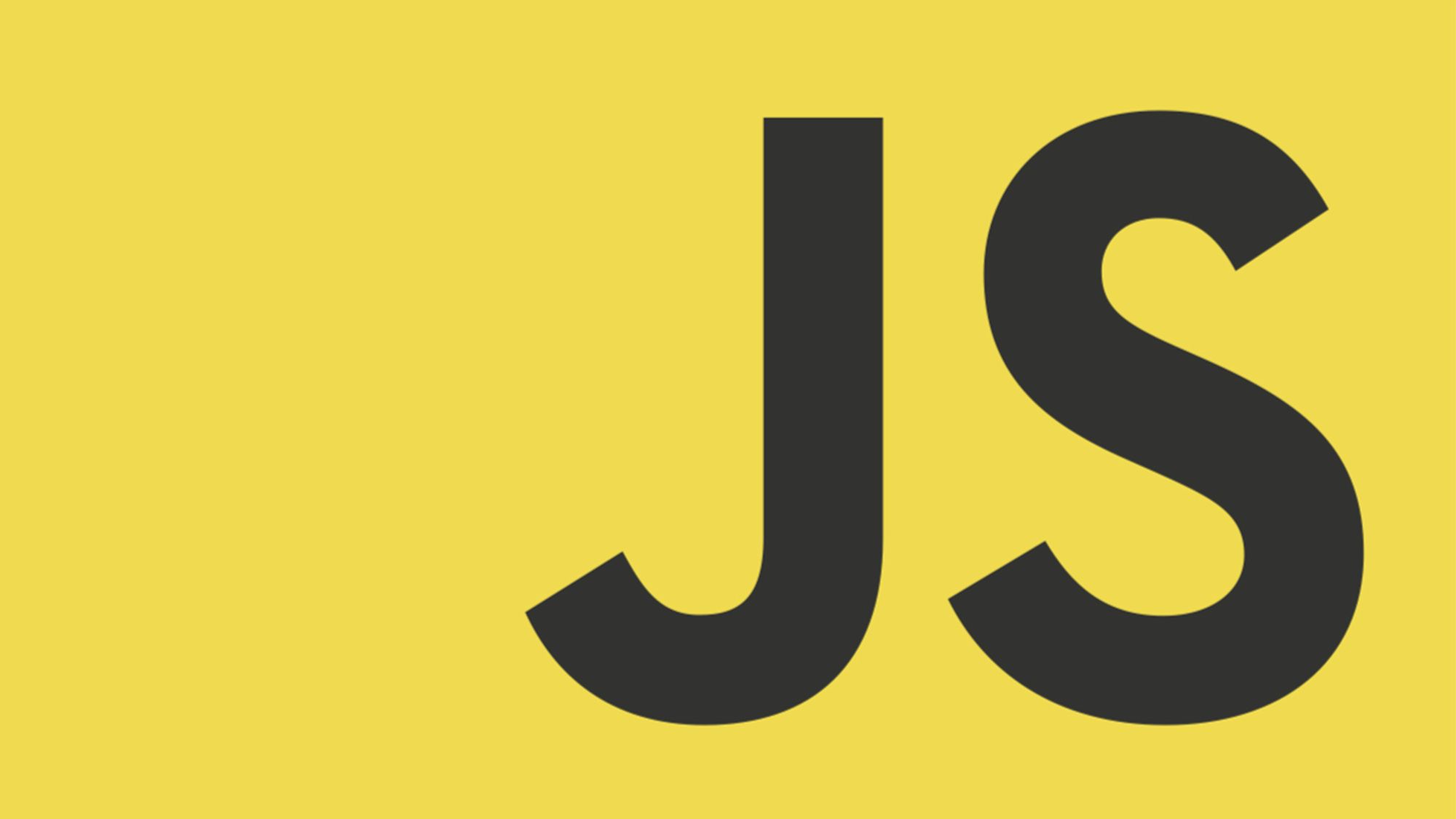
Dynamic actions are great, but sometimes I just want to code Javascript by hand in my code editor.
In the official APEX documentation, very little is said about APEX Javascript events.
All we know is that apexbeforerefresh
and apexafterrefresh
exist, but how can we use them? And are there more of these?
To my knowledge, here's a list of all APEX Javascript Events:
- apexwindowresized
- apexafterclosedialog
- apexafterhide
- apexaftershow
- apexbeforerefresh
- apexrefresh
- apexafterrefresh
- apexbeforepagesubmit
- apexpagesubmit
Here are some usage examples:
/*
* @event apexwindowresized
* @description Occurs when the APEX window is resized.
*
* @param e.type returns the name of the event
*/
$(window).on("apexwindowresized", function(e) {
console.log(e.type);
});
/*
* @event apexafterclosedialog
* @description Occurs when a modal window is closed.
* Cancelling a modal window does not trigger this event.
*
* @param e.type returns the name of the event
* @param data.successMessage.text returns the success coming from
* the modal window
*/
$(document).on("apexafterclosedialog", function(e, data) {
console.log(e.type);
console.log(data.successMessage.text);
});
/*
* @event apexafterhide
* @description Occurs after an APEX Dynamic Action "Hide"
*
* @param e.type returns the name of the event
* @param e.target is a reference to the element that was hidden
*/
$(document).on("apexafterhide", function(e) {
console.log(e.type);
console.log(e.target);
});
/*
* @event apexaftershow
* @description Occurs after an APEX Dynamic Action "Show"
*
* @param e.type returns the name of the event
* @param e.target is a reference to the element that was shown
*/
$(document).on("apexaftershow", function(e) {
console.log(e.type);
console.log(e.target);
});
/*
* @event apexbeforerefresh
* @description Occurs before an APEX element is being refreshed
*
* @param e.type returns the name of the event
* @param e.target is a reference to the element that was refreshed
* @param id contains the id of the old element
*/
$(document).on("apexbeforerefresh", function(e, id) {
console.log(e.type);
console.log(e.target);
console.log(id);
});
/*
* @event apexrefresh
* @description Occurs during an APEX element is being refresh
*
* @param e.type returns the name of the event
* @param e.target is a reference to the element that was refreshed
*/
$(document).on("apexrefresh", function(e) {
console.log(e.type);
console.log(e.target);
});
/*
* @event apexafterrefresh
* @description Occurs after an APEX element is being refreshed
*
* @param e.type returns the name of the event
* @param e.target is a reference to the element that was refreshed
*/
$(document).on("apexafterrefresh", function(e) {
console.log(e.type);
console.log(e.target);
});
/*
* @event apexbeforepagesubmit
* @description Occurs before a page is being submit
*
* @param e.type returns the name of the event
* @param request contains the request that is passed along with the page submit
*/
$(document).on("apexbeforepagesubmit", function(e, request) {
console.log(e.type);
console.log(request);
});
/*
* @event apexpagesubmit
* @description Occurs during a page is being submit
*
* @param e.type returns the name of the event
* @param request contains the request that is passed along with the page submit
*/
$(document).on("apexpagesubmit", function(e, request) {
console.log(e.type);
console.log(request);
});
Now of course the jQuery selector can vary with your application structure. For instance:
$(document).on("apexafterrefresh", function(e) {
...
});
can become the following for a better specificity:
$(".my-reports").on("apexafterrefresh", function(e) {
...
});
I still mostly use Dynamic Actions for simple event detection, but when an application gets bigger, I find that keeping those in a separate file is easier.